API list
Name | URL | Description |
---|---|---|
Upload files | https://api.zan.top/scr/v1/file/get-upload-link | Upload smart contract source code |
Create project | https://api.zan.top/scr/v1/project | Create a new project to run review task |
Stop project | https://api.zan.top/scr/v1/project/{projectId}/stop | Stop project's review task |
Retry project | https://api.zan.top/scr/v1/project/{projectId}/retry | Retry project's review task |
Query status | https://api.zan.top/scr/v1/project/{projectId}/status | Query project's review task status |
Query report detail | https://api.zan.top/scr/v1/project/{projectId}/report | Query project's report details |
Basic Usage Instructions
- You should get an Access Key before you start.
- Access Key is generated from SCR console by yourself. The following picture shows how to genenrate Access Key.
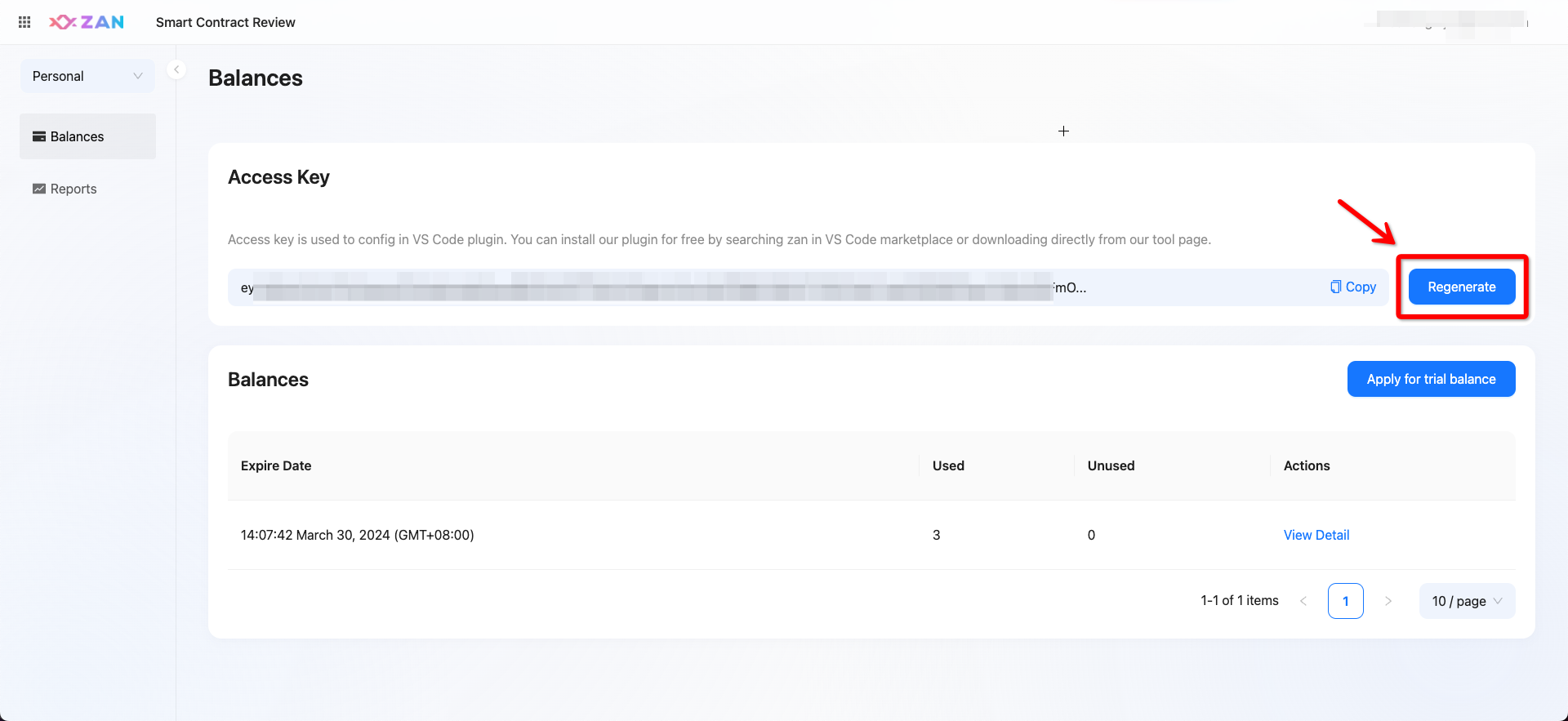
- Access Key should be added to the header of your request. Here are some examples about how to use Access Key. You can check API reference for more examples.
curl --request POST \
--url https://api.zan.top/project \
--header 'Authorization: Bearer {Your Access Key}' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"projectName": "demo",
"serviceType": "Express",
"compilerVersion": "0.8.2",
"srcForm": "FILE",
"ifSofiAudit": "false"
}
'
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"projectName\":\"demo\",\"serviceType\":\"Express\",\"compilerVersion\":\"0.8.2\",\"srcForm\":\"FILE\",\"ifSofiAudit\":\"false\"}");
Request request = new Request.Builder()
.url("https://api.zan.top/project")
.post(body)
.addHeader("accept", "application/json")
.addHeader("Authorization", "Bearer {Your Access Key}")
.addHeader("content-type", "application/json")
.build();
Response response = client.newCall(request).execute();
package main
import (
"fmt"
"strings"
"net/http"
"io"
)
func main() {
url := "https://api.zan.top/project"
payload := strings.NewReader("{\"projectName\":\"demo\",\"serviceType\":\"Express\",\"compilerVersion\":\"0.8.2\",\"srcForm\":\"FILE\",\"ifSofiAudit\":\"false\"}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("Authorization", "Bearer {Your Access Key}")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
const options = {
method: 'POST',
headers: {
accept: 'application/json',
Authorization: 'Bearer {Your Access Key}',
'content-type': 'application/json'
},
body: JSON.stringify({
projectName: 'demo',
serviceType: 'Express',
compilerVersion: '0.8.2',
srcForm: 'FILE',
ifSofiAudit: 'false'
})
};
fetch('https://api.zan.top/project', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));